Introduction: React developers often grapple with maintaining consistency and reusability in their interfaces, especially in sprawling applications. The Atomic Design methodology presents a systematic approach to tackle these challenges by deconstructing interfaces into their most basic elements, ensuring a harmonious and scalable UI ecosystem.

The Concept of Atomic Design: Atomic Design introduces a hierarchy of components, starting with the simplest units:
Atoms: These are the foundational building blocks of an interface, such as buttons and input fields. Consider the simplicity and versatility of Material-UI's components, which embody the atomic elements in React.
Molecules: When atoms combine, they form molecules. A search bar, integrating an input field and a button, exemplifies a molecule – a functional unit that leverages the combined properties of its atomic constituents.
Organisms: Organisms are complex UI components made of groups of molecules and possibly atoms. A website header, with its logo, navigation links, and search bar, represents an organism. GitHub's clean and functional header is a prime example.
Templates: Templates provide a structural context for organisms and molecules, like a wireframe that outlines where UI elements should be placed. Bootstrap and Ant Design offer a plethora of templates that demonstrate effective layouts for various interfaces.
Pages: The final level, pages, are instances of templates filled with actual content. They show how a template can be adapted to different content types, such as a blog homepage or an e-commerce product page.
Visual Elements: Incorporating diagrams or infographics can greatly enhance the comprehension of the Atomic Design hierarchy. Tools like Adobe XD or Sketch can be employed to create these visuals, offering a clear, graphical representation of the relationship between atoms, molecules, organisms, templates, and pages.
Real-World Examples: To anchor these concepts in reality, let's delve into real-world applications:
Atoms: Material-UI's Button component is an excellent atom example, encapsulating React's principles in a simple UI element.
Molecules: A search bar, blending Material-UI's Input and Button atoms, showcases a molecule's functionality.
Organisms: Spotify's header, with its combination of logo, navigation links, and search functionality, demonstrates an organism's complexity and utility.
Templates and Pages: The use of Ant Design templates to structure a dashboard, and then populating it with real content to create distinct pages, illustrates the practical application of templates and pages.
Benefits of Atomic Design with React: Atomic Design's modularity, reusability, and scalability are particularly advantageous in the React ecosystem. Tools like Storybook exemplify how React components can be documented and visualized at each level of Atomic Design, facilitating a more organized and efficient development process.
Step-by-Step Implementation Guide: To implement Atomic Design in React, start by identifying your UI's atoms and progressively build up to molecules, organisms, templates, and pages. Utilize tools like Storybook to document and prototype your components, ensuring consistency and reusability. GitHub repositories hosting example projects can serve as valuable resources for practical insights and inspiration.
Just to help with few examples -
Atoms
Atoms are the fundamental, indivisible components of your UI, such as buttons, input fields, and icons.
Naming Convention: Prefix the component name with Atom to indicate its atomic nature.
React Component Examples:
AtomButton.jsx: A basic button component. const AtomButton = ({ children, onClick }) => <button onClick={onClick}>{children}</button>;
AtomInput.jsx: A basic text input component. const AtomInput = ({ value, onChange }) => <input type="text" value={value} onChange={onChange} />;
Molecules
Molecules are groups of atoms bonded together to perform a specific function, like a search bar or a form field with a label.
Naming Convention: Prefix the component name with Molecule to indicate its molecular structure.
React Component Examples:
MoleculeSearchBar.jsx: Combines an AtomInput and an AtomButton. const MoleculeSearchBar = ({ query, onQueryChange, onSearch }) => ( <div> <AtomInput value={query} onChange={onQueryChange} /> <AtomButton onClick={onSearch}>Search</AtomButton> </div> );
Organisms
Organisms are complex UI components composed of multiple molecules and/or atoms, such as a site header.
Naming Convention: Prefix the component name with Organism to reflect its complexity.
React Component Examples:
OrganismHeader.jsx: Might include a logo (AtomIcon), navigation (MoleculeNavigation), and a search bar (MoleculeSearchBar). const OrganismHeader = ({ navItems }) => ( <header> <AtomIcon name="logo" /> <MoleculeNavigation items={navItems} /> <MoleculeSearchBar /> </header> );
Templates
Templates define the overarching structure or layout of a page but do not include the actual content.
Naming Convention: Prefix the component name with Template to indicate that it provides a template or layout.
React Component Examples:
TemplateStandardPage.jsx: Defines the layout for a standard page, including a header, footer, and content area. const TemplateStandardPage = ({ children }) => ( <div> <OrganismHeader /> <main>{children}</main> <OrganismFooter /> </div> );
Pages
Pages are the realization of templates filled with specific content, representing the final UI that users interact with.
Naming Convention: Typically named after their function or content within the application, without a specific prefix, to differentiate them from the component-based hierarchy.
React Component Examples:
HomePage.jsx: Implements the TemplateStandardPage with specific content for the home page. const HomePage = () => ( <TemplateStandardPage> <HeroSection /> <FeaturedProducts /> </TemplateStandardPage> );
Using this explicit naming convention, each component's role within the Atomic Design framework is immediately clear, streamlining development and maintenance processes within your React application.
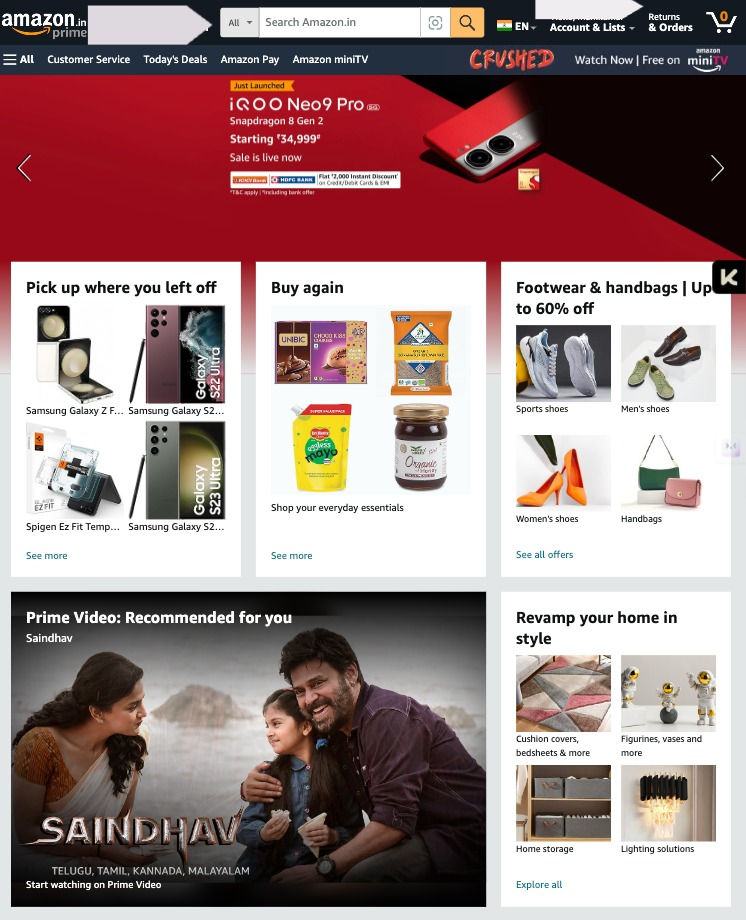
Atoms:
These are the basic building blocks of the page.
Text: Various text elements like product names, prices, and descriptions.
Icons: Small, symbolic images like the cart icon, user profile, and search magnifying glass.
Buttons: Clickable elements labeled "See more," "Buy again," or the navigation arrows on the carousel.
Input fields: The search bar at the top where users can type queries.
Molecules:
Molecules are groups of atoms combined to function as a unit.
Product Tile: A combination of a product image (atom), product name (atom), and price (atom).
Search Bar: The combination of the text input field (atom) and search icon/button (atom).
Organisms:
Organisms are complex UI components made up of molecules and possibly atoms.
Navigation Bar: The top bar with the Amazon logo (atom), search bar (molecule), location change (molecule), and user account links (molecule).
Product Carousel: A collection of product tiles (molecules) with navigation arrows (atoms) to scroll through items.
Templates:
A template defines the structure of the page and how organisms and molecules are laid out.
Page Layout: The overall layout showing the placement of the navigation bar (organism), main carousel (organism), and various sections for products and advertisements (organisms).
Pages:
Pages are specific instances of templates filled with actual content and data.
Homepage: The final rendered page that a user interacts with, filled with real products, real promotions (like the banner for the iQOO Neo9 Pro), and personalized content (like the "Pick up where you left off" section).
Using React, each of these levels would be constructed using components that correspond to atoms, molecules, organisms, and templates. The page itself is the composition of these components with actual data passed down via props or managed through state or context, creating the final interactive interface seen by the user.
コメント